Sending web-based text messages has never been easier thanks to Amazon SNS (Simple Notification Service). Whatever messaging you need SNS has you covered!
We’re going to run over three messaging scenarios: sending from AWS Console, sending from code, and sending account management messages on behalf of AWS Cognito.
Some quick pointers before continuing:
- Only 100 SMS messages are included in AWS free tier so review pricing before putting these into any form of production.
- This article only addresses SMS messaging and assumes some basic AWS knowledge. If you have questions on other resources mentioned below let me know in the comments!
Sending Texts from AWS Console
Open the AWS Console and Navigate to Amazon SNS. From there make sure you are in a region where the Text Messaging (SMS) tab is available — I’m using Oregon (us-west-2) below.
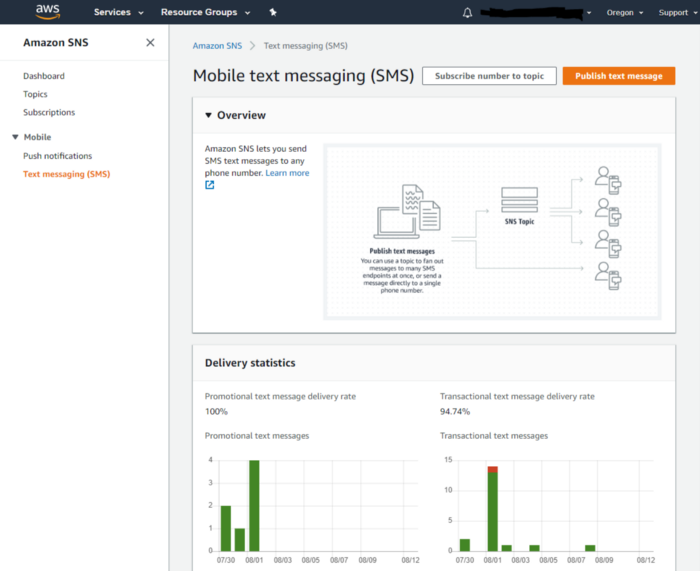
Once there go ahead and click on Publish text message.
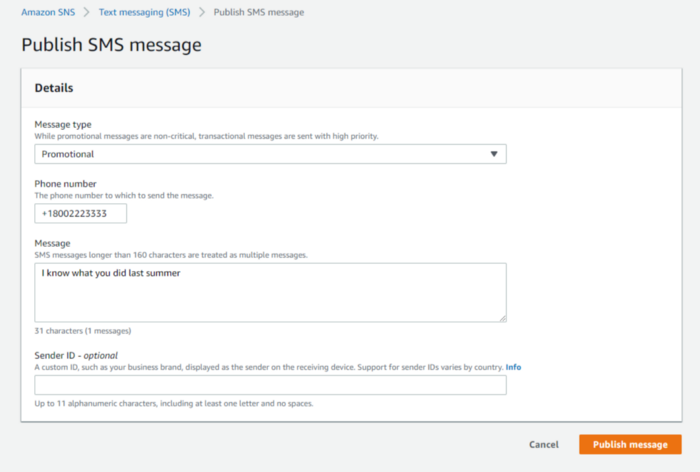
Enter the desired phone number in country code format, write your message, and click Publish message. That’s it!
This method isn’t realistic for production but can help with sending one-off messages and debugging: if you app messaging fails but they still send from console you have a better idea of where the break point is.
Sending Texts from Code
The easiest way to contact SNS is with the AWS SDK. Once you have the desired phone number and message you can submit them like so:
const AWS = require('aws-sdk');
testFunction = async(phoneNumber, message) => {
const SMSparams = {
Message: message, /* required */
PhoneNumber: phoneNumber
};
const publishTextPromise = new AWS.SNS({ apiVersion:
'2010-03-31' }).publish(SMSparams).promise();
await publishTextPromise.then( data => {
console.log("MessageID is " + data.MessageId);
}).catch( err => {
console.error(err, err.stack);
});
}
}
And… that’s not working!?
Remember that whatever’s running your code needs permission to access SNS, be it your live web page or an AWS Lambda.
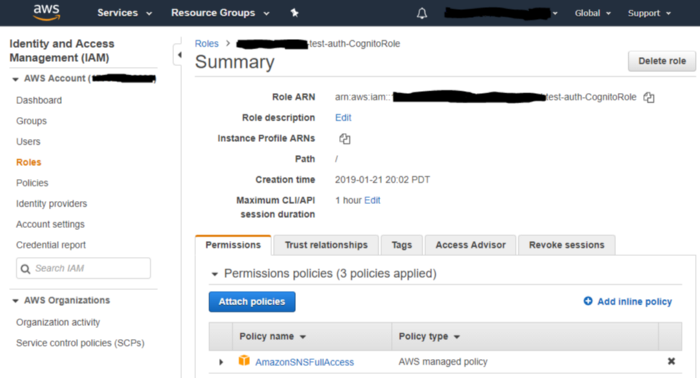
Find or create an IAM role (Identity and Access Management) for your executing resource to use. In my example I’m using test-auth-CognitoRole. Select Attach policies, find a valid SNS policy, and then attach it by clicking (you guessed it!) Attach Policy.
In this particular case I’ve selected AmazonSNSFullAccess to cover all my bases. Feel free to use or a create a policy with more granular access to SNS as needed.
Once this policy is connected your code resource should successfully send SMS messages 🎉
Sending Texts from Cognito (MFA, Logins, and More)
If you want to add SMS messaging to your multi-factor authentication (MFA) and/or login processes you’re in luck! Assuming you already have a user pool set up for your application head over to AWS Cognito and select the user pool you’d like to edit.
Navigate to MFA and verifications to enable MFA and then select the SMS method you’d like asa second factor. Below I’ve required MFA for my users, configured their MFA with SMS text messaging, and am verifying their phone number:

From there you can go to Message customizations on the left and edit messages to your heart’s desire. Now when a user tries to log in they should receive an SMS with your custom message.
Be sure to update your app so that users can enter this code! The example signup process uses AWS amplify so MFA can be accessed as such:
import { Auth } from 'aws-amplify';
const mfaSignup = (params) => {
return new Promise((resolve, reject) => {
Auth.confirmSignIn(params.user, params.code, params.mfaType)
.then(data => resolve(data))
.catch(err => reject(err));
});
},
That’s all folks!
Sending SMS messages may feel opaque at first but thankfully require minimal maintenance long-term. If you’ve found the above useful there are plenty of other resources that detail more complex use cases and tools— if you want to get fancy you can try including SMS rules in your Cognito CloudFormation template or schedule automatic SMS messaging using timed Lambda functions.